How to Create Stardew Valley Mods?
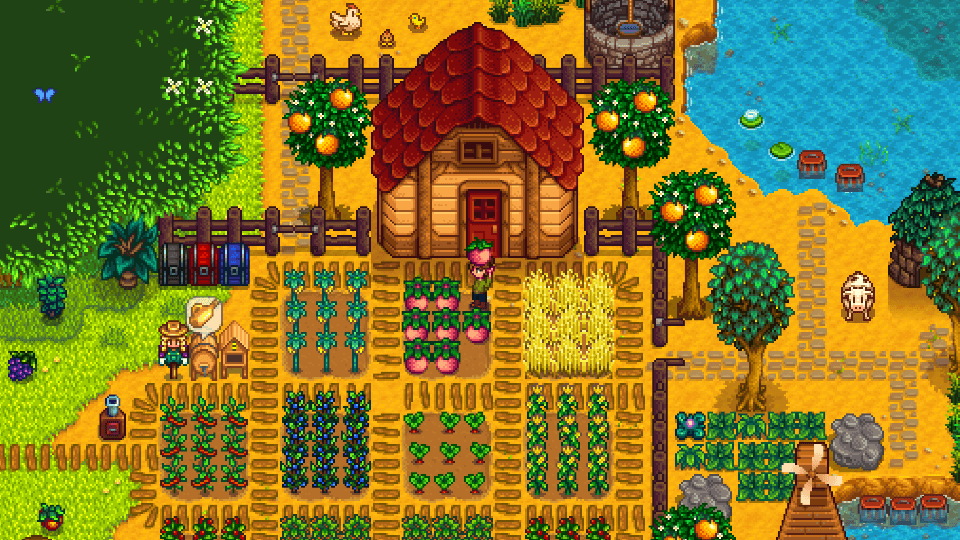
How to Create Stardew Valley Mods?
What is a SMAPI mod?
A SMAPI mod extends the game by employing the SMAPI modding API. The mod is able to provide response to whatever takes place in the game (for example, if something, some object, is added to the world), it can run the code at regular intervals (that means, for instance, a one-time-per-update tick), and modify the game’s assets, data, and so on. C# is used to write SMAPI mods, using the .NET Framework. Stardew Valley goes for XNA/MonoGame for the game logic (that includes screen drawing, user input, and so on).
Why do mods use SMAPI?
SMAPI can do so much! For instance, SMAPI can…
- Load your mod, which is great, because game code mods are simply impossible without SMAPI.
- Supply APIs and events that enable you to interact with the game in various ways that were not available before. More simple APIs are available for game asset/data alternations, player configuration, translation, reflection and other functions. They are described further in the guide.
- Rewrite your mod so that it becomes compatible with other platforms once it’s loaded. This way you can write mod code and not frett about the differences among the Linux/Mac/Windows versions of the game.
- Rewrite your so that it can be updated. One of the functions of SMAPI is to detect and repair a broken mod code, which usually is caused by a game update.
- Intercept errors. In the unfortunate event of your mod crashing or causing an error, SMAPI can quickly intercept the error, display the relevant details in the console window, and in most cases the game will be recovered automatically. That is very good, because you can be sure your mod won’t crash the game by accident, and error troubleshooting becomes much more simple.
- Inform you about updates. SMAPI Always lets players know if a new version of a mod is already available.
- Enable compatibility checks. SMAPI detects incompatible mods and disables tehm before any problems arise, so players are safe and so are their games.
Can I make a mod?
Absolutely. With the help of this guide, you will learn how to create a simple mod step-by-step. It’s much easier than you think – simply follow the guidelines and soon you will have your first mod.
Don’t worry if you haven’t done any programming before: many mod developers begin with very insignificant programming experience or none at all. You can keep learning as you go, however, it’s important to get the basics right at first. Don’t bite off more than you can chew; it’s better if you begin with a small mod and take your time to see how it works. Do not get overwhelmed from the start, it is really more simple than it seems. The modding community is here to help you, so don’t hesitate to contact them if you have questions!
If you already know how to do programming, it will be even easier for you. Programming experience in C# or Java is the best, but any programming knowledge will prove helpful. If you do not know much (or anything) about C#, it’s a good idea to look through the Learning C# references below to learn the most important stuff.
Can I make a mod without SMAPI?
You can. A number of SMAPI mods support ‘content packs’, these packs allow you to provide JSON text files, images, and so on used by them. Let’s say, you could choose to use Content Patcher to alter the game’s images and data, and there will be no need to do any programming whatsoever. The remaining part of this guide talks about how to make a new SMAPI mod; for content packs, you can check out the documentation for the mod that will read it.
Where can I get help?
You can always seek help with the Stardew Valley modding community. Do not hesitate to ask for help in #modding on the Stardew Valley Discord.
Get started
Learn C#
These mods are written in C#, so it will definitely be useful to become familiar with it first. There is no need to remember everything, but understanding the basics, such as fields, methods, variables, and classes will prove to be helpful in the future.
Here are some good resources for you:
- C# Quickstarts is great to learn the basics of C# , it‘s got interactive examples.
- C# Fundamentals for Absolute Beginners is a very good video guide tyhat takes you through C# step by step in a very comprehensible manner. You get to understand the basics as well as fairly complex functions.
Requirements
Before you take the first step:
- Get acquainted with the Player Guide. The rest of this guide is much more comprehensible for those who are already familiar with using mods.
- Install Stardew Valley.
- Install SMAPI.
- Install the IDE (integrated development environment).
- If you have Linux: install MonoDevelop.
- If you have Mac: install Visual Studio 2017 for Mac. (The same as MonoDevelop, just a different brand name.)
- If you have Windows: install Visual Studio 2017 Community. You will need to specify workloads, so just enable .NET Desktop Development.
If you do not know Visual Studio 2017 (on Windows/Mac) or MonoDevelop (on Linux), Modding:IDE reference is good for understanding how to do all the important stuff that will be necessary for this guide.
Create a basic mod
Quick start
If you think you have sufficient experience and can skip the tutorial, check out a quick summary of this section:
expand for quick start |
Create the project
A SMAPI mod is a library of compilations (DLL), its entry method gets called by SMAPI, therefore we begin by setting it up.
- Go to Visual Studio 2017 or MonoDevelop.
- Create a solution using a project of a .NET Framework class library (learn how to create a project). You have to be careful to select .NET Framework, not .NET Core or .NET Standard.
- Swap the target framework to .NET Framework 4.5, 4.5.1, or 4.5.2 to get the top compatibility (learn how to swap target framework).
- Reference the Pathoschild.Stardew.ModBuildConfig NuGet package (learn how to add the package).
- Once the package is installed, reference the Visual Studio/MonoDevelop.
Add the code
The next step is to add some code that SMAPI will run.
- Delete the Class1.cs or MyClass.cs file.
- Add a C# class file called ModEntry.cs to your project.
- Put this code in the file (replace YourProjectName with the name of your project):
4. using System;5. using Microsoft.Xna.Framework;6. using StardewModdingAPI;7. using StardewModdingAPI.Events;8. using StardewModdingAPI.Utilities;9. using StardewValley;10. 11.namespace YourProjectName12.{13. /// <summary>The mod entry point.</summary>14. public class ModEntry : Mod15. {16. /*********17. ** Public methods18. *********/19. /// <summary>The mod entry point, called after the mod is first loaded.</summary>20. /// <param name=”helper”>Provides simplified APIs for writing mods.</param>21. public override void Entry(IModHelper helper)22. {23. helper.Events.Input.ButtonPressed += this.OnButtonPressed;24. }25. 26. 27. /*********28. ** Private methods29. *********/30. /// <summary>Raised after the player presses a button on the keyboard, controller, or mouse.</summary>31. /// <param name=”sender”>The event sender.</param>32. /// <param name=”e”>The event data.</param>33. private void OnButtonPressed(object sender, ButtonPressedEventArgs e)34. {35. // ignore if player hasn’t loaded a save yet36. if (!Context.IsWorldReady)37. return;38. 39. // print button presses to the console window40. this.Monitor.Log($”{Game1.player.Name} pressed {e.Button}.”);41. }42. }43.}
Here’s an explanation of how the code works:
- using X; (see using directive) this is what provides classes in that namespace in your code.
- namespace YourProjectName (see namespace keyword) gives the definition of the scope for your mod code. This is something Visual Studio or MonoDevelop will add automatically when you add a file.
- public class ModEntry : Mod (see class keyword) creates your mod’s main class, and subclasses SMAPI’s Mod class. SMAPI will detect your Mod subclass and then Mod grants you access to SMAPI’s APIs.
- public override void Entry(IModHelper helper) is the method SMAPI calls once the mod is loaded. The helper offers easy access to a number of SMAPI’s APIs.
- helper.Events.Input.ButtonPressed += this.OnButtonPressed; adds an ‘event handler’ when an event triggered by a pressed button occurs. This means, that when a button is pressed (the helper.Events.Input.ButtonPressed event), SMAPI calls your this.OnButtonPressed method. Check events in the SMAPI reference to get more info.
Add your manifest
The function of the mod manifest is to inform SMAPI about your mod.
- Add a file called manifest.json to your project.
- Paste the following code into the file:
3. {4. “Name”: “<your project name>”,5. “Author”: “<your name>”,6. “Version”: “1.0.0”,7. “Description”: “<One or two sentences about the mod>”,8. “UniqueID”: “<your name>.<your project name>”,9. “EntryDll”: “<your project name>.dll”,10. “MinimumApiVersion”: “2.10.0”,11. “UpdateKeys”: []12.}
- Instead of the <…> placeholders enter the correct info. Make sure you leave no <> symbols!
You will find it listed in the console output at the time of the game launch. To learn more,check out the manifest docs.
Try your mod
- Try to build the project.
If all of your create the project steps were done with no mistakes, this will put your mod into the game’s Mods folder. - Now the game can be run through SMAPI.
For now, the mod will simply send a message to the console window every time you press a key in the game.
Troubleshoot
If the tutorial mod doesn’t work, try the following:
- Review the steps listed above to and see if you didn’t miss anything.
- See if you have for error messages, they often explain what the problem is:
- If you have Visual Studio, click Build > Rebuild Solution and see the Output pane or Error list.
- If you have MonoDevelop, click on Build > Rebuild All and give it a few minutes. After that, click on the “Build: XX errors, XX warnings” bar, and look at the XX Errors and Build Output tabs.
- Check out the troubleshooting guide.
- If nothing works, you can seek help in the #modding in the Stardew Valley Discord.
Go further
SMAPI APIs
SMAPI offers a selection of APIs that you can use if you wish to accomplish more. Check SMAPI reference for more info.
Crossplatform support
SMAPI will automatically adapt your mod to make it compatible with Linux, Mac, and Windows. Still, keep these tips in mind to avoid problems:
- Use the crossplatform build config package and set up your project references automatically. It facilitates crossplatform compatibility and allows your code compilation on any platform.
- Use Path.Combine to construct file paths, don’t use hardcode path separators because they may not function on all platforms.
3. // ✘ Don’t do this! It will crash on Linux/Mac.4. string path = Helper.DirectoryPath + “\assets\asset.xnb”;5. 6. // ✓ This is OK7. string path = Path.Combine(Helper.DirectoryPath, “assets”, “asset.xnb”);
- Use Helper.DirectoryPath, it is not a good idea at this point to determine the mod path yourself.
9. // ✘ Don’t do this! It will crash if SMAPI rewrites the assembly (e.g. to update or crossplatform it).10.string modFolder = Assembly.GetCallingAssembly().Location;11. 12.// ✓ This is OK13.string modFolder = Helper.DirectoryPath;
Decompile the game code
When you proceed to more complex mods, you probably will need to see how the game code functions.
Here is how you can decompile the game code so that it becomes readable (keep in mind that it will not function fully because of decompiler limitations):
- Windows:
- Open StardewValley.exe in dotPeek.
- Right-click on Stardew Valley and select Export to Project. Opt for the default in order to create a decompiled project that you will be able to see in Visual Studio.
- Linux/Mac:
- Open StardewValley.exe in MonoDevelop via File > Open.
- Change Language to C#.
For unpacking the XNB data/image files, go to Modding:Editing XNB files.
If you are a passionate player of Stardew Valley, you definitely know that mods are a really great tool. Need an example? For instance, Stardew Valley mods make it possible for you to upgrade the game in any way you wish. And there is more – you can even develop Stardew Valley mods independently and have an even wider choice of options. Just if you are feeling a bit overwhelmed with possibilities, have a look at the tips we share with you about how to create Stardew Valley mods. You have so many options to upgrade your Stardew Valley, this is why it’s a truly fantastic opportunity, be sure to make use of it. There are plenty of mod types that suit almost everyone – the variety is truly impressive and exciting – from maps and buildings or various objects to trailers, trucks and different tools. Now it is possible for you to remake and customize your Stardew Valley. It’s even better because if you decide to share your achievements with other members of the Stardew Valley community, you can do that, so take your chance! See how you create Stardew Valley mods by yourself and enjoy the game even more than before!
I will create video how to create mods for Stardew Valley 😉